ES6 引入了 class
语法,使得面向对象编程(OOP)在 JavaScript 中变得更加直观和易于理解。尽管 class
语法在表面上看起来与传统的构造函数非常相似,但它们之间有一些关键的区别和改进。以下是 class
和构造函数的主要区别:
1. 语法简洁性和可读性
构造函数:使用函数声明或函数表达式来定义,语法相对冗长。
function Person(name, age) { this.name = name; this.age = age; } Person.prototype.sayHello = function() { console.log(`Hello, my name is ${this.name}`); };
Class:语法更加简洁和清晰,更接近其他面向对象编程语言。
class Person { constructor(name, age) { this.name = name; this.age = age; } sayHello() { console.log(`Hello, my name is ${this.name}`); } }
2. 方法和属性的定义
构造函数:方法必须显式地添加到原型链上,通常通过
Person.prototype.methodName
的方式。Person.prototype.greet = function() { console.log(`Hi, I'm ${this.name}`); };
Class:方法和属性可以直接在类体内定义,更直观。
class Person { greet() { console.log(`Hi, I'm ${this.name}`); } }
3. Getter 和 Setter
构造函数:需要手动在原型链上定义 getter 和 setter。
Object.defineProperty(Person.prototype, 'fullName', { get: function() { return `${this.firstName} ${this.lastName}`; } });
Class:直接在类体内使用
get
和set
关键字定义 getter 和 setter。class Person { constructor(firstName, lastName) { this.firstName = firstName; this.lastName = lastName; } get fullName() { return `${this.firstName} ${this.lastName}`; } }
4. 静态方法
构造函数:静态方法需要手动添加到构造函数本身。
Person.createAdmin = function(name, age) { const admin = new Person(name, age); admin.isAdmin = true; return admin; };
Class:使用
static
关键字定义静态方法。class Person { static createAdmin(name, age) { const admin = new Person(name, age); admin.isAdmin = true; return admin; } }
5. 继承
构造函数:继承需要通过显式地设置原型链来实现。
function Employee(name, age, jobTitle) { Person.call(this, name, age); this.jobTitle = jobTitle; } Employee.prototype = Object.create(Person.prototype); Employee.prototype.constructor = Employee;
Class:使用
extends
关键字实现继承,更加简洁。class Employee extends Person { constructor(name, age, jobTitle) { super(name, age); this.jobTitle = jobTitle; } }
6. Super 调用
构造函数:在子构造函数中调用父构造函数需要显式地使用
call
或apply
。Employee.call(this, name, age);
Class:使用
super
关键字调用父构造函数和方法,语法更加简洁。super(name, age);
总结
ES6 的 class
语法提供了一种更加简洁、直观和易于理解的方式来定义和使用类。它不仅减少了样板代码,还提高了代码的可读性和可维护性。尽管 class
语法在底层仍然是基于原型链实现的,但它为 JavaScript 开发者提供了一种更接近传统面向对象编程的体验。
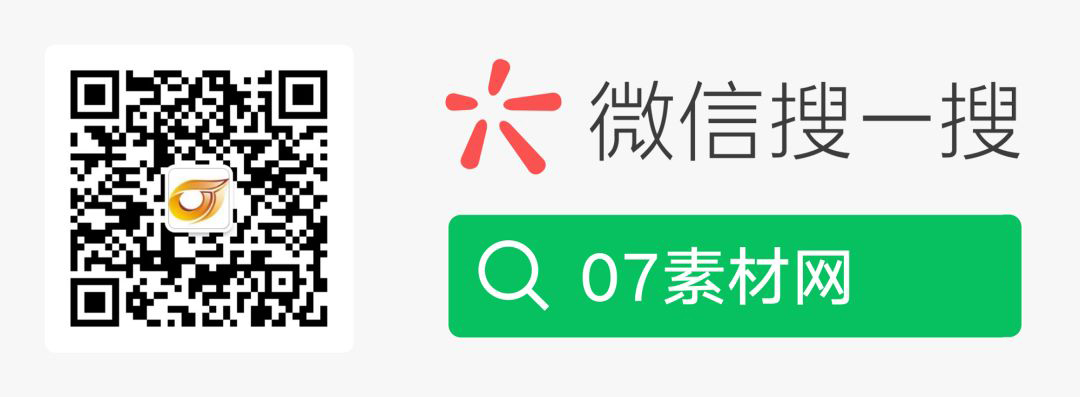